How to learn C++
The programming language C++ is essential to the area of system programming. This C++ tutorial will introduce you to some of the basics of C++, so that even beginners can get started.
What are some common uses of C++?
C++ is an object-oriented programming language and is suited to a variety of use cases. It is primarily used in system programming, thanks to its efficiency. The popular database management systems MongoDB and Apple OSX are written in C++. Essentially, C++ is a good choice for projects where resources are limited.
The language is also suited to programming different kinds of apps, for example, for creating user interfaces or even games.
What are the differences between C, C++ and C#?
If you’re interested in learning C++, you’ve probably also encountered C and C#. C forms the foundation of C++ and C#. It contains fewer elements than C++ and C# and is a procedural and imperative programming language. C has been used for system programming and hardware-oriented programming since the 1970s, due to its high portability.
C++ is an extension of C that supports object-oriented programming. C# is then an extension of C++, which is why it’s also known as C++++. Even though C++ and C# are both object-oriented programming languages that are based on C, they’re by no means identical. C++ gives programmers more freedom and is also more portable.
With ad-free webspace from IONOS, you can view all your web projects online. It can also serve as storage space for your most important projects.
What do you need to start learning C++?
To truly learn C++, you’ll need to write and compile your own C++ program. In most cases, it will suffice to get a text editor of your choosing and write code in it. Then you can translate your .cpp files into an executable program using a compiler. Depending on which operating system you’re using, you can use the compiler in the terminal or download one.
Alternatively, you can use an integrated development environment (IDE). The advantages of an IDE are clear. For one, you can test and compile your program right in the IDE. They also offer helpful features like syntax highlighting. Debugging is also a lot easier in a development environment. There are a variety of different IDEs that support C++, for example, Visual Studio and CLion.
If you’re just starting to learn how to program, it can also be helpful to take a look at some external resources that clearly explain the basic concepts in C++. You’ll find a number of videos on YouTube that can help you learn C++. The Simplilearn channel offers a quick 30-minute introduction in their video “C++ Tutorial For Beginners”.
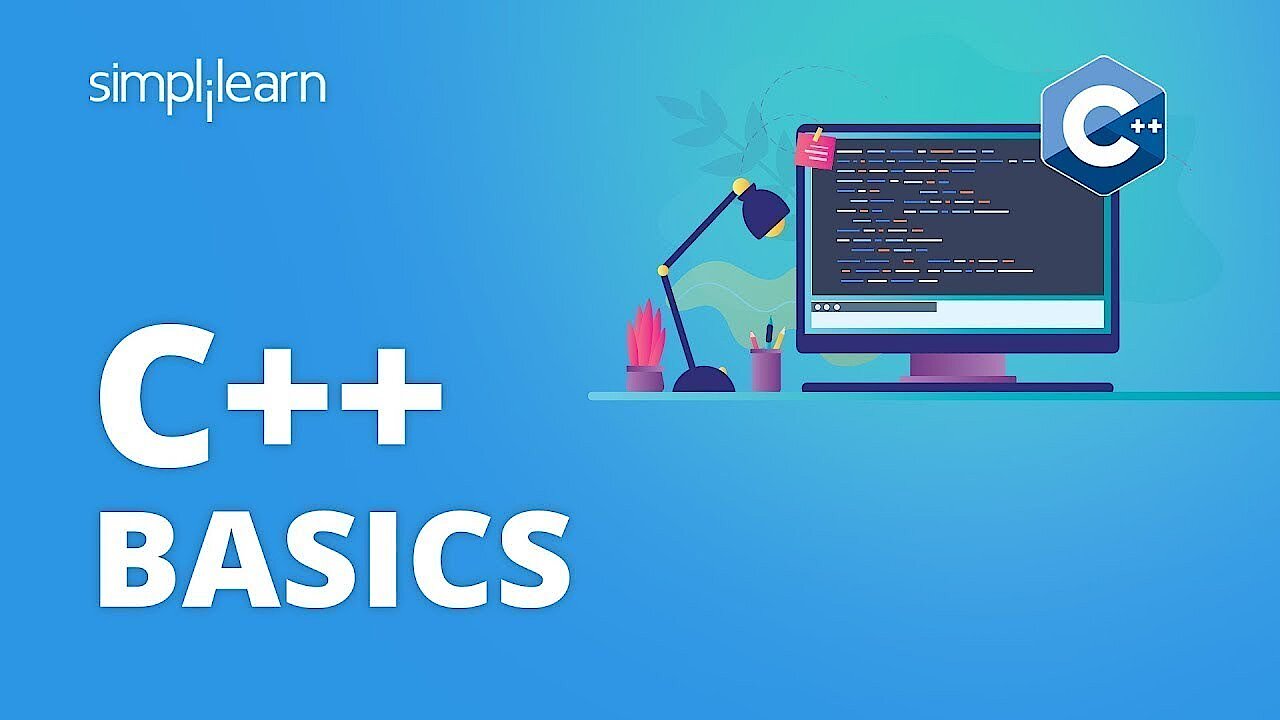
Aside from YouTube, Microsoft’s C++ documentation is a great source of information.
How to write the most important control structures in C++
The syntax of C++ is very similar to the syntax of C. Most C++ operators also exist in C. So if you already have knowledge of C, it will be considerably easier for you to learn C++.
Just like every other programming language, C++ distinguishes between different control structures that need to be formulated in the correct syntax for the code to be compiled without errors. Other elements, like comments, also follow a specific syntax.
C++ is also case sensitive, meaning that it distinguishes between capital and lowercase letters. Additionally, sets of statements need to be enclosed in curly brackets. Unlike in languages like Python, the brackets mean that indentation isn’t necessary, though it does make code significantly easier to read. Every statement in C++ ends with a semicolon.
Are you interested in learning other programming languages? Check out our other beginner-friendly tutorials:
Comments
If you want to add notes to your code that should be ignored by the compiler, you can use comments. There are a couple different ways to make comments in C++:
// A single line comment
/* A comment that takes up
more than one line */
C++Data types
Similar to other programming languages, there are different data types in C++. Each of these data types is introduced with a different keyword. If you want to create a variable, you’ll need to explicitly assign that data type to it.
// Integer
int number = 5;
// Floating point
float decnumber = 0.5f;
// String
string word = "Hello!";
// Character
char letter = 'D';
// Boolean
bool truthvalue = true;
C++These are just a few of the most frequently used data types. This list is by no means exhaustive.
If-else statements
As in most other programming languages, you can use if-else statements in C++ if part of the code should only be executed when a condition is met. The syntax used for this in C++ is similar to that in C and Java.
if (condition) {
// Code that will be executed if condition is fulfilled
} else {
// Code that will be executed if condition is not fulfilled
}
C++For and while loops
Loops are another fundamental programming concept that you’ll find in C++. If you want to repeat part of your code as long as a certain condition is being met, use a while loop.
int i = 0;
while (i <= 5) {
cout << i << "\n";
i++;
}
C++In the above code, a variable of the integer data type has been created, which will save a whole number. The variable with the name “i” is assigned the value 0. The while loop will run until the variable’s value exceeds 5. Each time the loop is executed, the current value of the variable is output and increased by 1 (incremented).
You can achieve the same things using a for loop in C++, also known as a counting loop. In for loops, a variable is set and automatically changed with each run through the loop.
for (int i = 0; i <= 5; i++) {
cout << i << "\n";
}
C++Switch statement
The switch statement in C++ offers an elegant solution for distinguishing between different conditions and executing code based on that.
int input;
cin >> input;
switch (input) {
case 0:
cout << "A 0 was entered";
break;
case 1:
cout << "A 1 was entered";
break;
case 2:
cout << "A 2 was entered";
break;
default:
cout << "Another number was entered";
break;
}
C++In the above code, the person who is executing the program is asked to enter an integer that is saved as a variable under the name “input”. In the switch statement that follows, the value of the variable is used to distinguish between different parts of the code: If 0, 1 or 2 was entered, that will be the output. Otherwise, the statement under “default” will be executed.